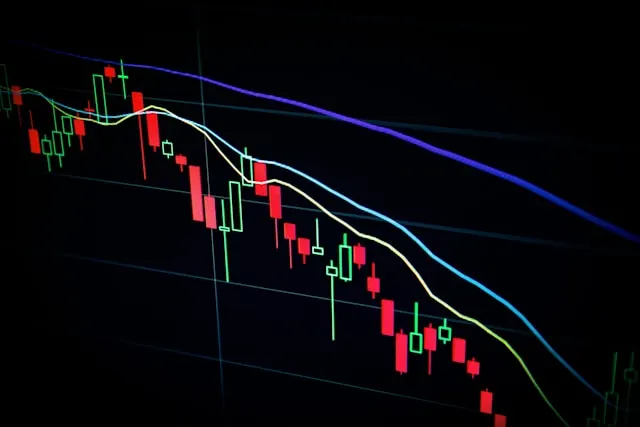
Photo by Maxim Hopman on Unsplash
Experience the 2008 financial meltdown through code and data visualization. Code the 2008 Crisis
Introduction
The 2008 financial crisis was a pivotal moment in global economic history, marked by the collapse of major financial institutions and unprecedented market volatility. By simulating this crisis using Python, you can gain deeper insights into market dynamics and the factors that led to such a significant downturn. This tutorial will guide you through building a stock market simulator that replicates the 2008 crisis, leveraging historical data and Python’s powerful libraries.
1. Understanding the 2008 Financial Crisis
Before diving into the code, it’s essential to grasp the events that unfolded during the crisis:
- Subprime Mortgage Collapse: High-risk mortgage lending practices led to widespread defaults.
- Lehman Brothers Bankruptcy: The fall of this major investment bank triggered panic in global markets.
- Stock Market Plunge: Major indices like the S&P 500 experienced significant declines.
By simulating these events, we can observe how such factors influence stock prices and market behavior.
2. Setting Up Your Python Environment
Ensure you have the following Python libraries installed:
bashpip install pandas numpy matplotlib yfinance
These libraries will help in data retrieval, manipulation, and visualization.
3. Fetching Historical Stock Data
We’ll use the yfinance
library to obtain historical stock data for major banks affected during the crisis.
import yfinance as yf
import pandas as pd
import matplotlib.pyplot as plt
# List of bank tickers
tickers = ['BAC', 'C', 'GS', 'JPM', 'MS', 'WFC']
# Fetch data from 2006 to 2009
start_date = '2006-01-01'
end_date = '2009-12-31'
# Dictionary to store data
stock_data = {}
for ticker in tickers:
data = yf.download(ticker, start=start_date, end=end_date)
stock_data[ticker] = data['Adj Close']
This code retrieves adjusted closing prices for each bank, providing a foundation for our simulation.GitHub
4. Visualizing Stock Performance
Let’s visualize the stock performance of these banks during the crisis.
pythonCopyEdit# Combine all data into a single DataFrame
combined_data = pd.DataFrame(stock_data)
# Plotting
plt.figure(figsize=(14, 7))
for ticker in tickers:
plt.plot(combined_data[ticker], label=ticker)
plt.title('Bank Stock Prices During the 2008 Financial Crisis')
plt.xlabel('Date')
plt.ylabel('Adjusted Close Price')
plt.legend()
plt.grid(True)
plt.show()
This plot will illustrate the decline in stock prices, highlighting the severity of the crisis.
5. Simulating the Crisis with Monte Carlo
To model the uncertainty and volatility during the crisis, we’ll implement a Monte Carlo simulation.
import numpy as np
def monte_carlo_simulation(start_price, days, mu, sigma, simulations):
results = []
for _ in range(simulations):
prices = [start_price]
for _ in range(days):
price = prices[-1] * np.exp((mu - 0.5 * sigma**2) + sigma * np.random.normal())
prices.append(price)
results.append(prices)
return results
# Parameters
start_price = combined_data['JPM'].iloc[0]
days = 252 # Approximate trading days in a year
mu = combined_data['JPM'].pct_change().mean()
sigma = combined_data['JPM'].pct_change().std()
simulations = 100
# Run simulation
simulated_prices = monte_carlo_simulation(start_price, days, mu, sigma, simulations)
# Plotting
plt.figure(figsize=(14, 7))
for simulation in simulated_prices:
plt.plot(simulation, color='gray', alpha=0.1)
plt.title('Monte Carlo Simulation of JPM Stock Price')
plt.xlabel('Days')
plt.ylabel('Price')
plt.grid(True)
plt.show()
This simulation provides a range of possible future stock prices, reflecting the uncertainty during the crisis.
6. Analyzing the Results
By comparing the simulated data with actual historical prices, we can assess the accuracy and effectiveness of our model. This analysis can offer insights into risk management and investment strategies during volatile periods.
7. Conclusion
Simulating the 2008 financial crisis using Python offers a hands-on approach to understanding market dynamics during extreme events. By leveraging historical data and statistical models, we can gain valuable insights into risk assessment and decision-making in the financial world.
Related reads: Simulating ancient romes hyperinflation with python